In Laravel, you can use aggregate functions to perform calculations on database records. Laravel’s query builder provides methods to work with aggregate functions, such as count
, sum
, avg
, max
, and min
. These methods allow you to retrieve aggregated data from your database tables.
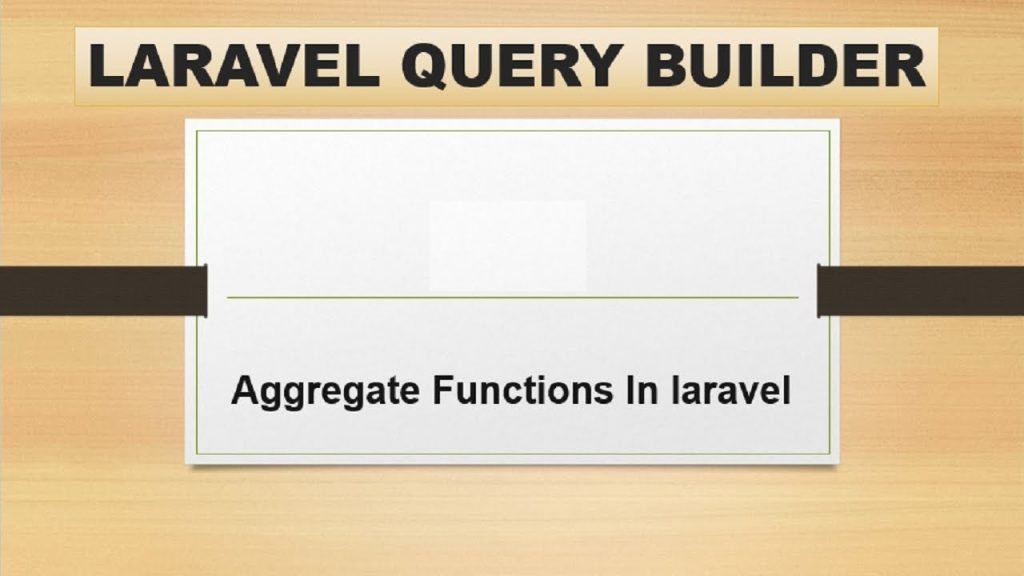
Here’s an example of how to use aggregate functions in Laravel using the query builder:
1. Count the number of records:
$count = DB::table('table_name')->count();
2. Calculate the sum of a column:
$sum = DB::table('table_name')->sum('column_name');
3. Calculate the average of a column:
$average = DB::table('table_name')->avg('column_name');
4. Find the maximum value in a column:
$maxValue = DB::table('table_name')->max('column_name');
5. Find the minimum value in a column:
$minValue = DB::table('table_name')->min('column_name');
You can also combine aggregate functions with query conditions and other query builder methods to perform more specific calculations and filtering.