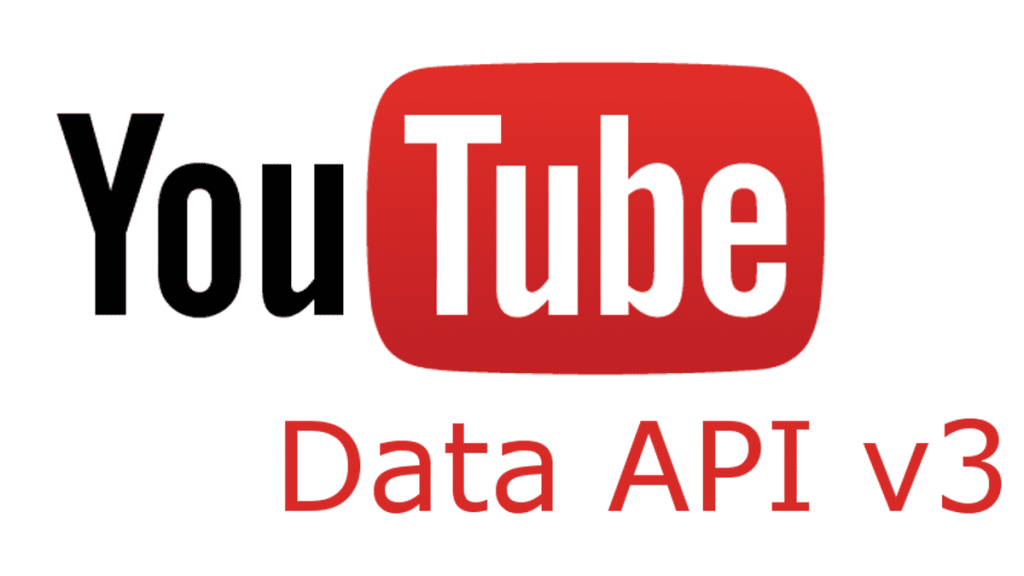
In Controller make a function:-
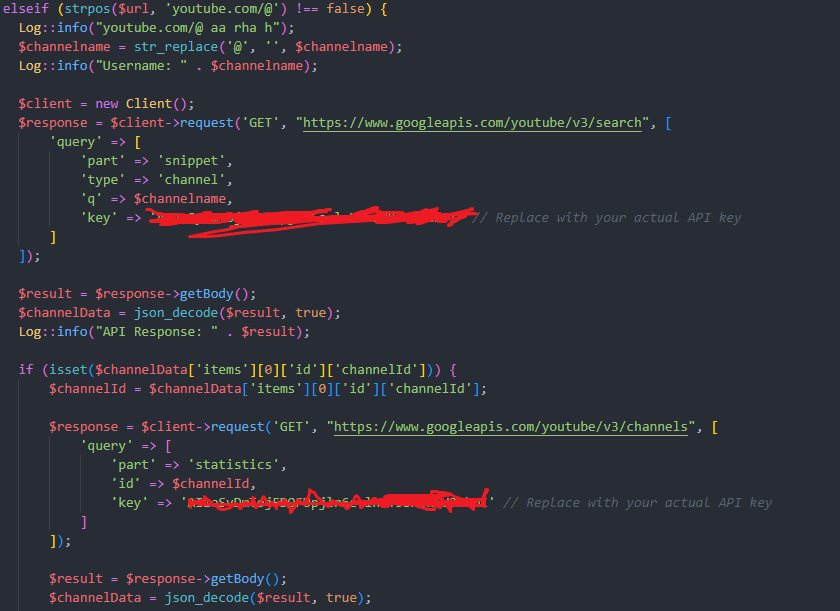
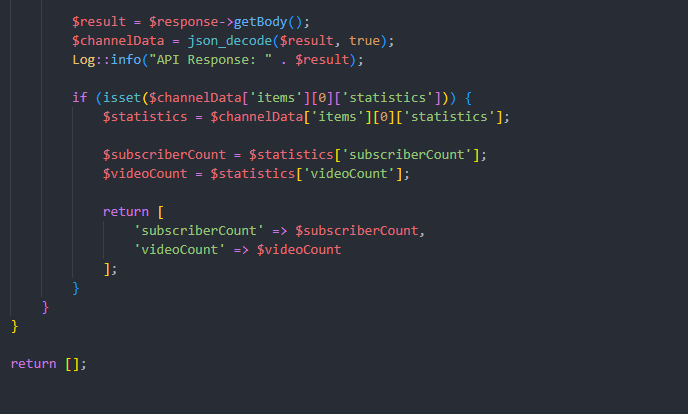
CODE
if (strpos($url, 'youtube.com/channel/') !== false) {
$channelIdArr = $this->getYouTubeXMLUrl($url, true);
if ($channelIdArr && isset($channelIdArr['id'])) {
$channelId = $channelIdArr['id'];
$client = new Client();
$response = $client->request('GET', "https://www.googleapis.com/youtube/v3/channels", [
'query' => [
'part' => 'statistics',
'id' => $channelId,
'key' => ''
]
]);
$result = $response->getBody();
$channelData = json_decode($result, true);
if (isset($channelData['items'][0]['statistics'])) {
$statistics = $channelData['items'][0]['statistics'];
$subscriberCount = $statistics['subscriberCount'];
$videoCount = $statistics['videoCount'];
return [
'subscriberCount' => $subscriberCount,
'videoCount' => $videoCount
];
}
}
} elseif (strpos($url, 'youtube.com/@') !== false) {
Log::info("youtube.com/@ aa rha h");
$channelname = str_replace('@', '', $channelname);
Log::info("Username: " . $channelname);
$client = new Client();
$response = $client->request('GET', "https://www.googleapis.com/youtube/v3/search", [
'query' => [
'part' => 'snippet',
'type' => 'channel',
'q' => $channelname,
'key' => '' // Replace with your actual API key
]
]);
$result = $response->getBody();
$channelData = json_decode($result, true);
Log::info("API Response: " . $result);
if (isset($channelData['items'][0]['id']['channelId'])) {
$channelId = $channelData['items'][0]['id']['channelId'];
$response = $client->request('GET', "https://www.googleapis.com/youtube/v3/channels", [
'query' => [
'part' => 'statistics',
'id' => $channelId,
'key' => '' // Replace with your actual API key
]
]);
$result = $response->getBody();
$channelData = json_decode($result, true);
Log::info("API Response: " . $result);
if (isset($channelData['items'][0]['statistics'])) {
$statistics = $channelData['items'][0]['statistics'];
$subscriberCount = $statistics['subscriberCount'];
$videoCount = $statistics['videoCount'];
return [
'subscriberCount' => $subscriberCount,
'videoCount' => $videoCount
];
}
}
}
return [];
Output :-
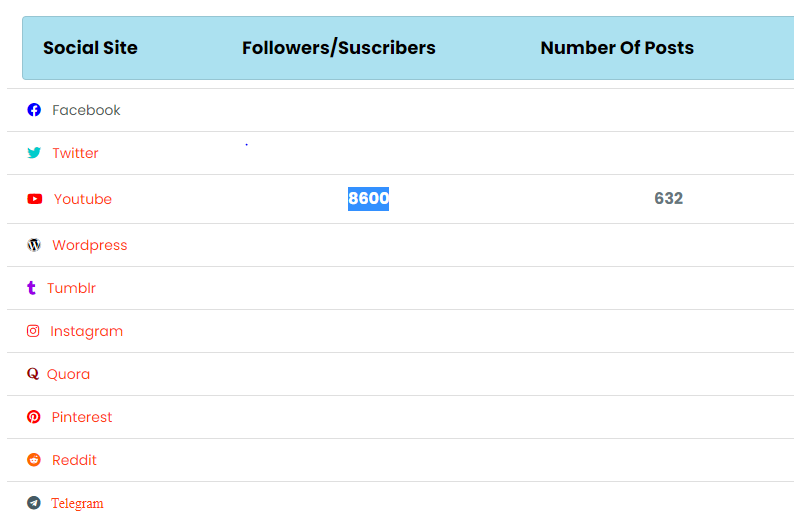