Introduction
In this tutorial, we will learn how to create a zip file and download it in Laravel 10. We will explore the step-by-step process with a live example. Are you ready? Let’s dive in!
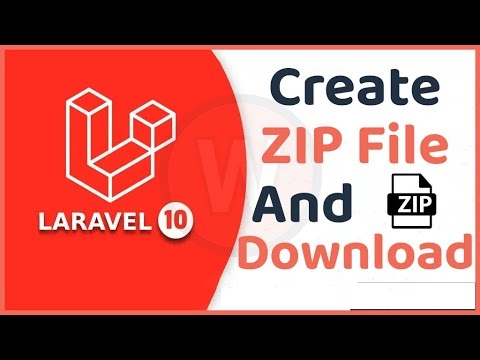
Step 1: Set up a Laravel project
First, let’s create a new Laravel project by running the following command in your terminal:
composer create-project laravel/laravel example-app
Step 2: Create a route
Next, let’s create a route in the web.php
file located in the routes
directory. Open the file and add the following code:
Route::get('/download-zip', [DownloadController::class, 'downloadZip']);
Step 3: Create a controller
Now, let’s create a controller called DownloadController
. Run the following command in your terminal:
Open the newly created DownloadController.php
file in the app/Http/Controllers
directory and add the following code:
use ZipArchive;
class DownloadController extends Controller
{
public function downloadZip()
{
// Create a new zip archive
$zip = new ZipArchive;
// Path to the directory you want to compress
$directory = public_path('files');
// Path to the zip file you want to create
$zipFile = public_path('downloads/files.zip');
// Open the zip file for writing
if ($zip->open($zipFile, ZipArchive::CREATE) === TRUE) {
// Add all files from the directory to the zip file
$files = File::allFiles($directory);
foreach ($files as $file) {
$zip->addFile($file->getPathname(), $file->getBasename());
}
// Close the zip file
$zip->close();
}
// Download the zip file
return response()->download($zipFile);
}
}
Step 4: Create a view
php artisan make:view download
<!DOCTYPE html>
<html>
<head>
<title>Download Zip File</title>
</head>
<body>
<h1>Download Zip File</h1>
<a href="{{ url('/download-zip') }}">Click here to download the zip file</a>
</body>
</html>
Step 5: Update the route
Route::get('/download', function () {
return view('download');
});
Output:-
