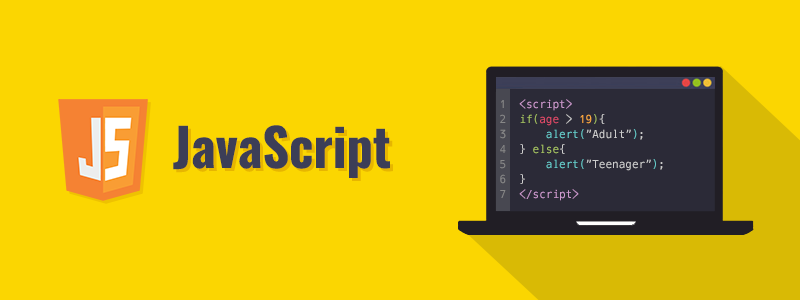
JavaScript is a scripting or programming language that allows you to implement complex features on web pages every time a web page does more than just sit there and display static information for you to look at displaying timely content updates, interactive maps, animated 2D/3D graphics, scrolling video jukeboxes, etc. you can bet that JavaScript is probably involved. It is first discovered by “Brenden Eich” in 1995, JavaScript is also known as ECMAScript. Currently the update version is ECMA6.
HTML is the markup language that we use to structure and give meaning to our web content, for example defining paragraphs, headings, and data tables, or embedding images and videos in the page.
CSS is a language of style rules that we use to apply styling to our HTML content, for example setting background colors and fonts, and laying out our content in multiple columns.
JavaScript is a scripting language that enables you to create dynamically updating content, control multimedia, animate images, and pretty much everything else. (Okay, not everything, but it is amazing what you can achieve with a few lines of JavaScript code.)
The three layers build on top of one another nicely. Let’s take a simple text label as an example.
Then we can add some CSS into the mix to get it looking nice:
And finally, we can add some JavaScript to implement dynamic behavior:
JavaScript can do a lot more than that — let’s explore what in more detail.
So what can it really do?
The core client-side JavaScript language consists of some common programming features that allow you to do things like:
- Store useful values inside variables. In the above example for instance, we ask for a new name to be entered the store that name in a variable called name.
- Operations on pieces of text (known as “strings” in programming). In the above example we take the string “Player 1: ” and join it to the name variable to create the complete text label, e.g. ”Player 1: name”.
- Running code in response to certain events occurring on a web page. We used a click event in our example above to detect when the button is clicked and then run the code that updates the text label, and much more!
JavaScript running order
When the browser encounters a block of JavaScript, it generally runs it in order, from top to bottom. This means that you need to be careful what order you put things in. For example, let’s return to the block of JavaScript we saw in our first example:
Here we are selecting a heading (line 1), then attaching an event listener to it (line 3) so that when the heading is clicked, the myFunction() code block (lines 5–8) is run. The myFunction() code block (these types of reusable code blocks are called “functions”) asks the user for a new name, and then inserts that name into the paragraph to update the display.
If you swapped the order of the first two lines of code, it would no longer work, you’d get an error returned in the browser developer console, and the TypeError: element is undefined. This means that the element object does not exist yet, so we can’t add an event listener to it. This is a very common error — you need to be careful that the objects referenced in your code exist before you try to do stuff to them.
Interpreted versus compiled code
You might hear the terms interpreted and compiled in the context of programming. In interpreted languages, the code is run from top to bottom and the result of running the code is immediately returned. You don’t have to transform the code into a different form before the browser runs it. The code is received in its programmer-friendly text form and processed directly from that.
Compiled languages on the other hand are transformed (compiled) into another form before they are run by the computer. For example, C/C++ are compiled into machine code that is then run by the computer. The program is executed from a binary format, which was generated from the original program source code.
In case of JavaScript, It is a lightweight interpreted programming language. The web browser receives the JavaScript code in its original text form and runs the script from that. From a technical standpoint, most modern JavaScript interpreters actually use a technique called just-in-time compiling to improve performance; the JavaScript source code gets compiled into a faster, binary format while the script is being used, so that it can be run as quickly as possible. However, JavaScript is still considered an interpreted language, since the compilation is handled at run time, rather than ahead of time.
Server-side versus client-side code
You might also hear the terms server-side and client-side code, especially in the context of web development. Client-side code is code that is run on the user’s computer when a web page is viewed, the page’s client-side code is downloaded, then run and displayed by the browser. In this module we are explicitly talking about client-side JavaScript.
Server-side code on the other hand is run on the server, then its results are downloaded and displayed in the browser. Examples of popular server-side web languages include PHP, Python, Ruby, ASP.NET and… JavaScript! JavaScript can also be used as a server-side language, for example in the popular Node.js environment — you can find out more about server-side JavaScript in our Dynamic Websites – Server-side programming topic.
Dynamic versus static code
The word dynamic is used to describe both client-side JavaScript, and server-side languages, it refers to the ability to update the display of a web page/app to show different things in different circumstances, generating new content as required. Server-side code dynamically generates new content on the server, e.g. pulling data from a database, whereas client-side JavaScript dynamically generates new content inside the browser on the client, e.g. creating a new HTML table, filling it with data requested from the server, then displaying the table in a web page shown to the user. The meaning is slightly different in the two contexts, but related, and both approaches (server-side and client-side) usually work together.
A web page with no dynamically updating content is referred to as static, it more like just shows the same content all the time.
Summary
So there you go, your first step into the world of JavaScript. We’ve begun with just theory, to start getting you used to why you’d use JavaScript and what kind of things you can do with it. Along the way, you saw a few code examples and learned how JavaScript fits in with the rest of the code on your website, amongst other things.JavaScript may seem a bit daunting right now, but don’t worry in this course, we will take you through it in simple steps that will make sense going forward. In the next article, we will plunge straight into the practical, getting you to jump straight in and build your own JavaScript examples.
I hope you like this blog about JavaScript, Keep practising and exploring.
Thank You!!